JavaScript interview questions and their answers are essential for anyone preparing for interviews. In this compilation, we have gathered the top 10 frequently asked top 10 javascript interview questions that cater to both beginners and professionals. Whether you are new to JavaScript or an experienced developer, these questions will help you enhance your knowledge and excel in interviews. Let’s dive into the list of essential JavaScript interview questions!
What is JavaScript?
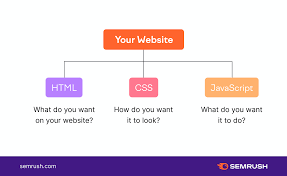
JavaScript is a versatile scripting language distinct from Java. Being object-based and lightweight, it serves as a cross-platform translated language. One of its primary applications lies in client-side validation. For web browsers, the embedded JavaScript Translator plays a crucial role in translating JavaScript code.
List some features of JavaScript

- JavaScript boasts several noteworthy features:
Lightweight: JavaScript’s efficiency lies in its lightweight nature, making it ideal for web development.
- Interpreted Programming Language: As an interpreted language, JavaScript allows for rapid development and debugging.
- Network-Centric Applications: JavaScript excels in building applications that rely on network interactions.
- Complementary to Java: JavaScript’s compatibility with Java enhances its versatility in various projects.
- Complementary to HTML: Working seamlessly with HTML, JavaScript enhances web pages’ interactivity and functionality.
- Open Source: As an open-source language, JavaScript benefits from a collaborative community and continuous improvement.
- Cross-Platform: JavaScript’s cross-platform capabilities enable its deployment on multiple devices and operating systems.
Who developed JavaScript, and what was the first name of JavaScript?
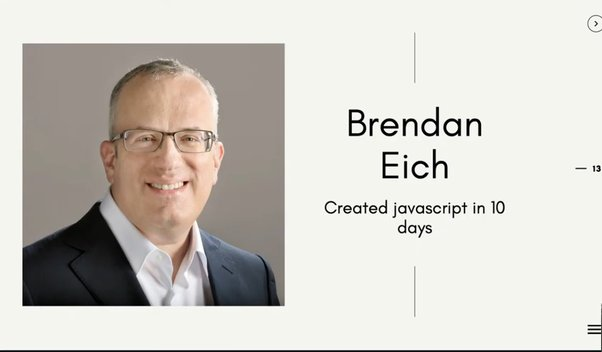
JavaScript, a scripting language widely used in web development, was created by Brendan Eich, a programmer at Netscape. In a remarkable feat, Eich developed this language in a mere ten days during September 1995. Initially named Mocha upon its release, the language underwent several name changes, eventually settling on JavaScript, previously known as Live Script.
List some of the advantages of JavaScript.
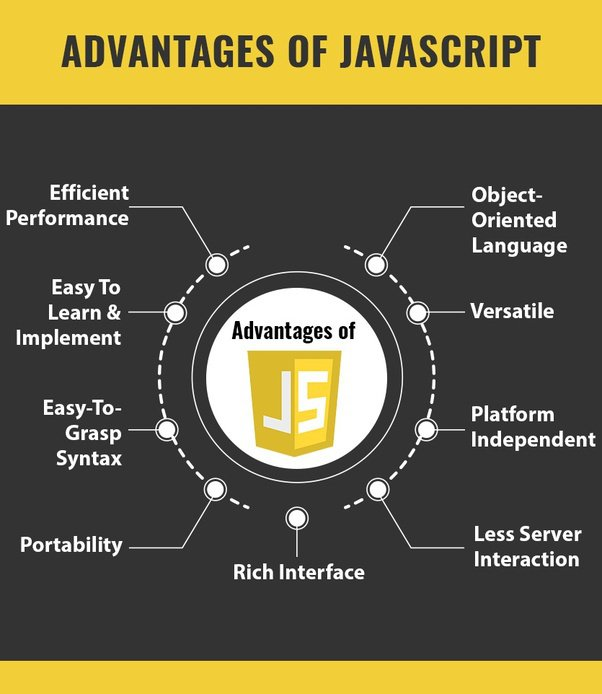
JavaScript offers a host of advantages that enhance web development:
- Reduced Server Interaction: JavaScript’s client-side capabilities minimize the need for frequent server interactions, resulting in improved performance.
- Instant Feedback: With JavaScript, websites can provide immediate feedback to visitors, leading to a more responsive and user-friendly experience.
- Enhanced Interactivity: Thanks to JavaScript’s dynamic nature, websites can achieve high levels of interactivity, engaging users in a more compelling manner.
- Richer Interfaces: JavaScript empowers developers to create visually appealing and feature-rich user interfaces, elevating the overall user experience.
List some of the disadvantages of JavaScript.
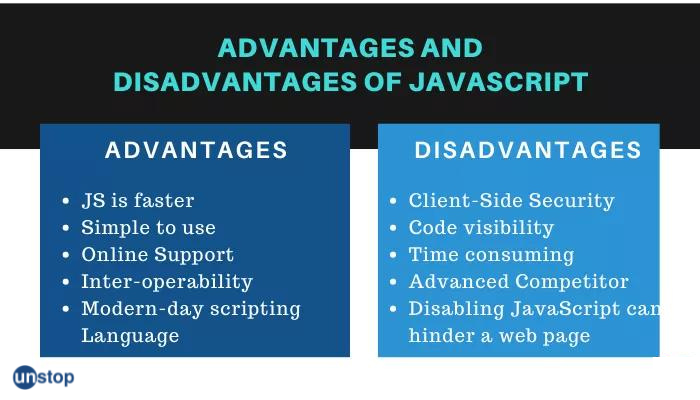
JavaScript does have some limitations that developers should be aware of:
- Lack of Multithreading Support: JavaScript lacks built-in support for multithreading, which can hinder performance in certain scenarios.
- Absence of Multiprocessing Support: Similarly, JavaScript does not provide native support for multiprocessing, limiting its ability to handle computationally intensive tasks efficiently.
- Restrictions on File Handling: JavaScript does not permit direct reading and writing of files on the user’s system due to security concerns.
- Limited Networking Capabilities: While JavaScript is extensively used for client-side interactions, it lacks robust built-in support for complex networking applications, which may require additional workarounds.
Define a named function in JavaScript.
A named function is a function that is given a specific name at the time of its definition. For instance:
function showMessage() {
document.writeln("Named Function");
}
showMessage();
Name the types of functions
Functions in JavaScript can be classified into two types:
- Named Functions: These functions are defined with a specific name at the time of declaration. For example:
function showMsg() {
document.writeln("Named Function");
}
showMsg();
In this case, “showMsg” is a named function, and when invoked, it will display “Named Function” on the web page.
- Anonymous Functions: Unlike named functions, anonymous functions are declared dynamically at runtime and do not have a specific name. For instance:
var display = function() {
document.writeln("Anonymous Function");
};
display();
Here, “display” is an anonymous function, and calling it will output “Anonymous Function.” Anonymous functions are often used as callbacks or in situations where a function is needed temporarily.
Define anonymous function
An anonymous function is a function without a name, defined dynamically at runtime using the function operator rather than a function declaration. Compared to function declarations, the function operator offers greater flexibility and can be seamlessly used in place of an expression. Here’s an example:
var display = function() {
alert("Anonymous Function is invoked");
};
display();
Can an anonymous function be assigned to a variable?
Certainly! In JavaScript, it is possible to assign an anonymous function to a variable. This capability allows you to create functions without explicitly naming them and store them in variables for later use. Here’s an example:
var myFunction = function() {
// Code for the anonymous function goes here
};
// You can then call the function using the variable name
myFunction();
In JavaScript what is an argument object?
In JavaScript, variables serve as placeholders that hold the values of arguments passed to functions. When a function is called, these variables act as containers for the provided arguments, allowing the function to work with the passed data effectively. Through this mechanism, JavaScript functions can receive input and process it to perform specific tasks or computations.